Java for Keyword
The Java for keyword is used to create a conditional statement loop called for loop which executes a set of statements in each iteration. See the syntax below:
Syntax
for(initialization(s); condition(s); counter_update(s);){ statements; }
- Initialization(s): Variable(s) is initialized in this section and executed for one time only.
- Condition(s): Condition(s) is defined in this section and executed at the start of loop everytime.
- Counter Update(s): Loop counter(s) is updated in this section and executed at the end of loop everytime.
Flow Diagram:
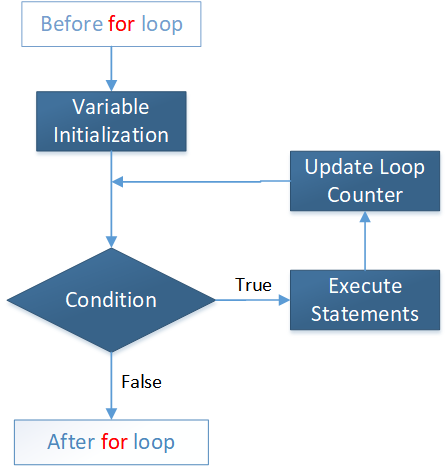
Example:
In the example below, the program continue to print variable called i from value 1 to 5. After that, it exits from the for loop.
public class MyClass { public static void main(String[] args) { for (int i = 1; i <= 5; i++){ System.out.println(i); } } }
The output of the above code will be:
1 2 3 4 5
Example
Multiple initializations, condition checks and loop counter updates can be performed in a single for loop. See the example below:
public class MyClass { public static void main(String[] args) { for (int i = 1, j = 100; i <= 5 || j <= 800; i++, j = j + 100){ System.out.println("i="+i+", j="+j); } } }
The output of the above code will be:
i=1, j=100 i=2, j=200 i=3, j=300 i=4, j=400 i=5, j=500 i=6, j=600 i=7, j=700 i=8, j=800
Java for-each Loop
Java for-each loop is used to traverse an array or a collection. In each iteration of the loop, current element is assigned to a user-defined variable and array/collection pointer is moved to next element which is processed in the next iteration. Please note that it works on element basis (not index basis).
Syntax
for(variable: array/collection){ statements; }
In the example below, the foreach loop is used on array called Arr which is further used to print each element of the array in each iteration.
public class MyClass { public static void main(String[] args) { int Arr[]={10, 20, 30, 40 ,50}; System.out.println("The Arr contains:"); for(int i: Arr) System.out.println(i); } }
The output of the above code will be:
The Arr contains: 10 20 30 40 50
❮ Java Keywords