AJAX - Tutorial
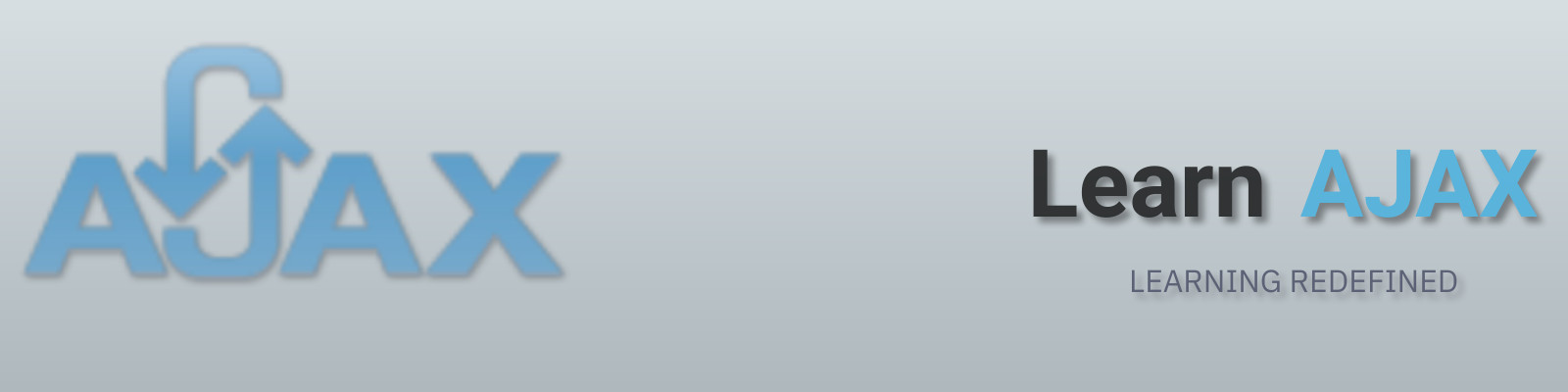
AJAX stands for Asynchronous JavaScript and XML. It is a set of web development techniques that uses various web technologies on the client-side to create asynchronous web applications. With Ajax, web applications can send and retrieve data from a server asynchronously (in the background) without interfering with the display and behavior of the existing page. By decoupling the data interchange layer from the presentation layer, Ajax allows web pages and, by extension, web applications, to change content dynamically without the need to reload the entire page.
About Tutorial
This tutorial is intended for students and professionals interested in studying basic and advanced concepts of AJAX. This tutorial covers all topics of AJAX which includes XMLHttpRequest, database operations, security, issues, browser support and examples. We believe in learning by examples therefore each and every topic is explained with lots of examples that makes you learn AJAX in a very easy way.
Along with this, almost all examples can be executed online which provides better understanding of the language and helps you to learn Ajax faster. Consider a simple example below which is for the illustration purpose and uses Ajax technique to change the content of a HTML div without interfering with the display and behavior of the current page.
<div id="demo"> <h1>The XMLHttpRequest Object</h1> <button type="button" onclick="loadInfo()">Change Content</button> </div> <script> function loadInfo() { var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { document.getElementById("demo").innerHTML = this.responseText; } }; xhttp.open("GET", "ajax_demo.txt", true); xhttp.send(); } </script>
The output of the above script will be:
The XMLHttpRequest Object
We will discuss about this example in detail in later section.
Prerequisite
Before continuing with this tutorial, you should have basic understanding of HTML and JavaScript.