Java.util package Tutorial
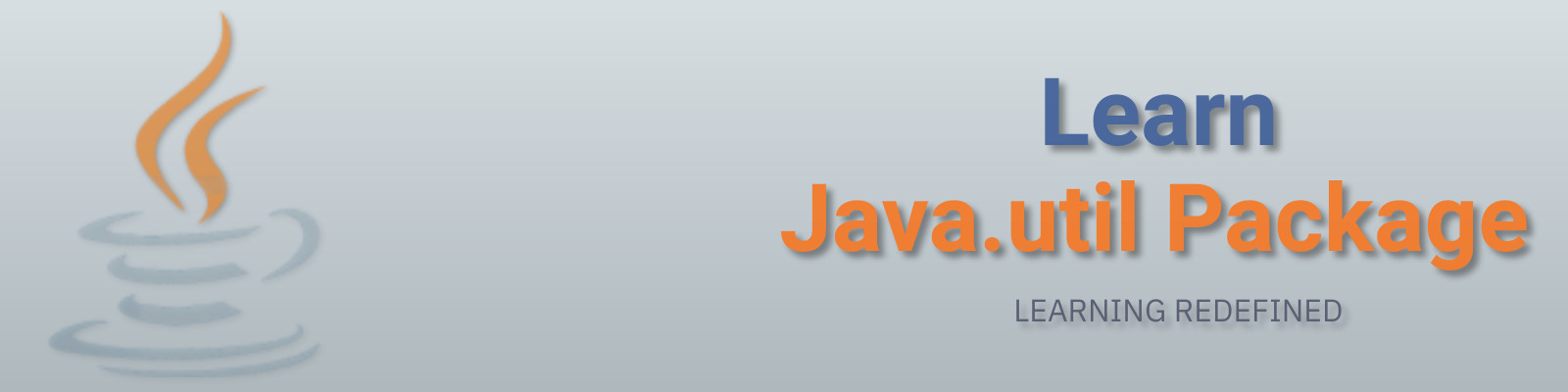
Java.util package contains the collections framework, legacy collection classes, event model, date and time facilities, internationalization, and miscellaneous utility classes (a string tokenizer, a random-number generator, and a bit array).
About Tutorial
This tutorial is intended for students and professionals interested in studying basic and advanced concepts of Java utility package. This tutorial covers introduction, declaration and methods of most commonly used Java classes present in Java utility package, for example - Array, ArrayDeque, ArrayList, BitSet, Calendar, Date, Dictionary, Time, Random, Scanner & Vector class. We believe in learning by examples therefore each and every topic is explained with lots of examples that makes you learn the topic in a very easy way. Along with this, almost all examples can be executed online which provides better understanding of the package and helps you to learn the concept faster. The classical example of printing the content of Java LinkedList is mentioned below for the illustration purpose.
import java.util.*; public class MyClass { public static void main(String[] args) { //creating a linkedlist LinkedList<Integer> MyList = new LinkedList<Integer>(); //populating linkedlist MyList.add(10); MyList.add(20); MyList.add(30); //printing linkedlist System.out.print("MyList contains: " + MyList); } }
The output of the above code will be:
MyList contains: [10, 20, 30]
Prerequisite
Before continuing with this tutorial, you should have basic understanding of Java programming language. This section contains various types of examples which are used for reference and requires basic understanding of Java language.