Matplotlib - Tutorial
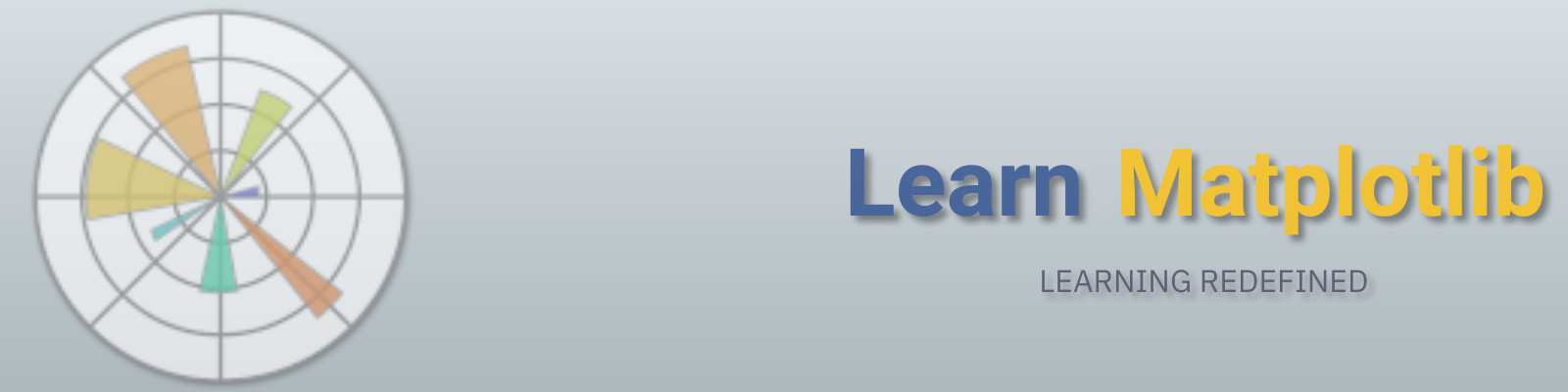
Matplotlib is a plotting library for the Python programming language used for data visualization. It provides an object-oriented API for embedding plots into applications using general-purpose GUI toolkits like Tkinter, wxPython, Qt, or GTK+. There is also a procedural "pylab" interface designed to closely resemble that of MATLAB, with the ability to use Python, and the advantage of being free and open-source. Matplotlib was originally written by John D. Hunter and is distributed under a BSD-style license.
About Tutorial
This tutorial is intended for software programmers interested in studying basic and advanced concepts of Matplotlib. This tutorial covers all topics of Matplotlib which includes figure class, axes class, multiplot, subplot, grid and different types of plots. We believe in learning by examples, therefore, each topic is explained with lots of examples that makes you learn Matplotlib in a very easy way. Along with this, almost all examples can be executed online which provides better understanding of the package and helps you to learn it faster. An example of contour plot of a circular plane is mentioned below for the illustration purpose:
import matplotlib.pyplot as plt import numpy as np xlist = np.linspace(-5.0, 5.0, 100) ylist = ylist = np.linspace(-5.0, 5.0, 100) X, Y = np.meshgrid(xlist, ylist) #creating circular plane Z = np.sqrt((X**2) + (Y**2)) fig,ax = plt.subplots(1,1) cb = ax.contourf(X, Y, Z) #Addind a colorbar to the plot fig.colorbar(cb) ax.set_title('Contour Plot') ax.set_xlabel('x (cm)') ax.set_ylabel('y (cm)') plt.show()
The output of the above code will be:
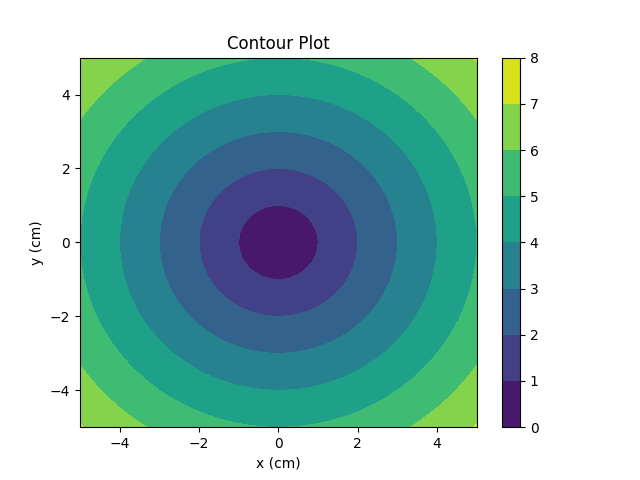
Prerequisite
A prior exposure of Python programming language would be an added advantage while following this tutorial. Matplotlib library uses most of its functionality from NumPy library. Therefore, it is recommended to go through the NumPy Tutorial before following this tutorial.