Matplotlib - 3D Surface Plot
A three-dimensional axes can be created by passing projection='3d' keyword to the axes creation routine. After creating 3D axes, matplotlib.Axes3D.plot_surface() function creates a surface plot. By default it will be colored in shades of a solid color, but it also supports color mapping by supplying the cmap argument.
Syntax
matplotlib.Axes3D.plot_surface(X, Y, Z, rcount, ccount, rstride, cstride, cmap)
Parameters
X, Y, Z |
Required. Specify Data values. |
rcount, ccount |
Optional. Specify maximum number of samples used in each direction. If the input data is larger, it will be downsampled (by slicing) to these numbers of points. Defaults to 50. |
rstride, cstride |
Optional. Specify downsampling stride in each direction. These arguments are mutually exclusive with rcount and ccount. If only one of rstride or cstride is set, the other defaults to 10. |
cmap |
Optional. Specify a colormap for the surface patches. |
Example: 3D surface plot of test data
In the example below, surface plot is drawn for the test data present within the package.
import matplotlib.pyplot as plt from mpl_toolkits.mplot3d import axes3d fig = plt.figure() ax = fig.add_subplot(111, projection='3d') ax.set_title('Surface Plot') #getting test data X, Y, Z = axes3d.get_test_data(0.05) #drawing surface plot cb = ax.plot_surface(X, Y, Z, color="green") plt.show()
The output of the above code will be:
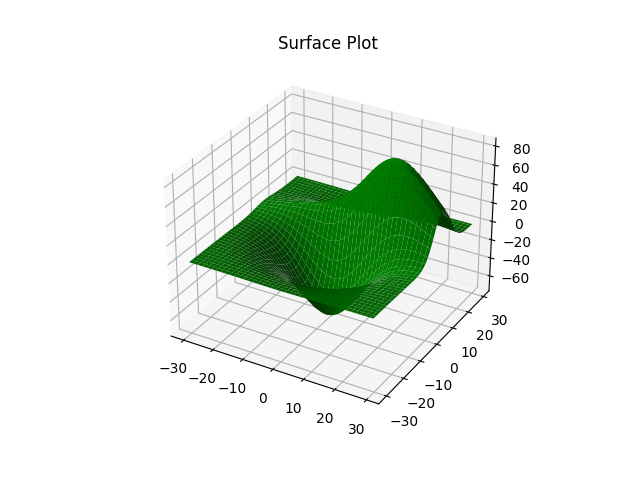
Example: 3D surface plot
Lets consider one more example. Here, the surface plot is drawn from user-defined 3D plane.
import matplotlib.pyplot as plt import numpy as np def f(x, y): return np.sin(np.sqrt(x ** 2 + y ** 2)) xlist = np.linspace(-6.0, 6.0, 100) ylist = ylist = np.linspace(-6.0, 6.0, 100) X, Y = np.meshgrid(xlist, ylist) #creating 3D plane Z = f(X, Y) fig = plt.figure() ax = fig.add_subplot(111, projection='3d') ax.set_title('Surface Plot') #drawing surface plot cb = ax.plot_surface(X, Y, Z) plt.show()
The output of the above code will be:
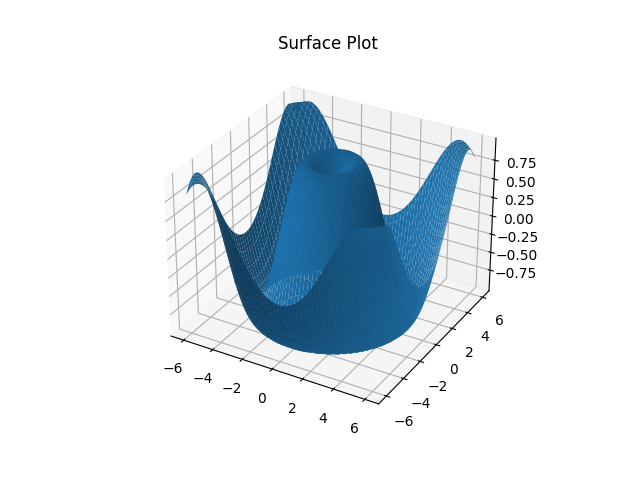