Matplotlib - Working with Images
The image module in Matplotlib package is used for working with images in Python. It provides functionalities required for loading, re-scaling and displaying image. The image module also includes two useful function which are imread() which is used to read images and imshow() which is used to display the image.
Example: read and display images
Let us assume, we have the following image:
In the example below, the program reads this image using the image.imread() function and displays the image using image.imshow() function.
import matplotlib.pyplot as plt import matplotlib.image as mpimg img = mpimg.imread('matplotlib.PNG') plt.imshow(img)
The output of the above code will be:
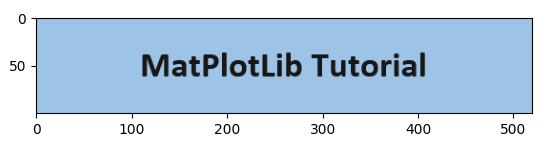
Example: importing image data into NumPy arrays
In the example below, the program reads this image and represents the image in array.
import matplotlib.pyplot as plt import matplotlib.image as mpimg img = mpimg.imread('matplotlib.PNG') print(img)
The output of the above code will be:
[[[0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] ... [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ]] [[0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] ... [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ]] [[0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] ... [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ]] ... [[0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] ... [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ]] [[0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] ... [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ]] [[0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] ... [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ] [0.6156863 0.7647059 0.9019608 1. ]]]
Example: applying pseudocolor to image plots
Pseudocolor can be a useful tool for enhancing contrast and visualizing your data more easily. This is especially useful when making presentations of your data using projectors - their contrast is typically quite poor.
Shape of the image can be represented as (height, width, mode) of the image, for colored image mode value is from 0 to 2 and for black and white image mode value is 0 and 1 only.
import matplotlib.pyplot as plt import matplotlib.image as mpimg img = mpimg.imread('matplotlib.PNG') lum_img = img[:, :, 0] plt.imshow(lum_img)
The output of the above code will be:
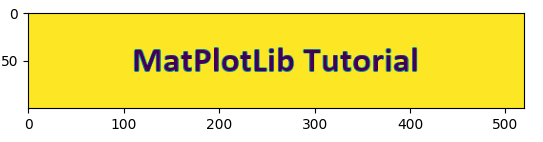
Now, with a luminosity (2D, no color) image, the default colormap is applied.
import matplotlib.pyplot as plt import matplotlib.image as mpimg img = mpimg.imread('matplotlib.PNG') lum_img = img[:, :, 0] plt.imshow(lum_img, cmap="hot")
The output of the above code will be:
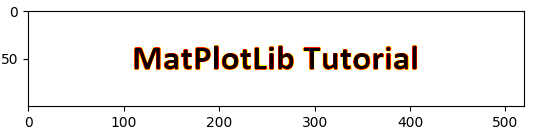
Example: displaying a portion of image plots
In the example below, the height of the image is 35 pixels which is displayed from 25th pixel, width is 150 pixels which is displayed from 100th pixel and the mode value is 1.
import matplotlib.pyplot as plt import matplotlib.image as mpimg img = mpimg.imread('matplotlib.PNG') modified_img = img[25:60, 100:250, 1] plt.imshow(modified_img)
The output of the above code will be:
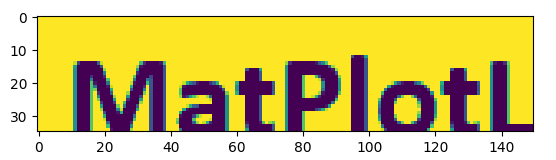