Matplotlib - Object-oriented API
At its core, Matplotlib is object-oriented. Matplotlib recommend directly working with the objects as it gives more control and customization of the plots. In object-oriented interface, a Figure can be divided into two different objects:
- Figure object
- Axes object
A Figure object can contain one or more axes objects. One axes represents one plot inside figure. Figure class and Axes class are discussed in detail in later sections.
Creating plot using Object-oriented API
Before using any matplotlib.pyplot function, first of all, the pyplot module is imported from Matplotlib as shown below:
import matplotlib.pyplot as plt
After importing the module, all functions inside Pyplot module can be used in the current script. To start with, a figure object can be added using below syntax. The figure object provides an empty canvas for the figure.
fig = plt.figure()
After that axes object is added to figure using add_axes() function. It requires a list containing four elements representing left, bottom, width and height receptively of the figure. Each element must be between 0 and 1.
ax = fig.add_axes([0,0,1,1])
Then, a graph can be plotted on the given axes using plot() function. We can also set title and axes labels of a plot.
#drawing the plot ax.plot(x,y) #setting title ax.set_title("sine wave") #setting x-axis label ax.set_xlabel('angle') #setting y-axis label ax.set_ylabel('sine')
Example: simple plot
Consider the example below where a simple of y = sin(x) is drawn using object-oriented API.
import matplotlib.pyplot as plt import numpy as np #creating an array of values between #0 to 10 with a difference of 0.1 x = np.arange(0, 10, 0.1) y = np.sin(x) #creating figure and axes objects fig = plt.figure() ax = fig.add_axes([0.15,0.1,0.8,0.8]) #formatting axes ax.set_title("Sine wave") ax.set_xlabel("x") ax.set_ylabel("y = sin(x)") #drawing the plot ax.plot(x, y) #displaying the figure plt.show()
The output of the above code will be:
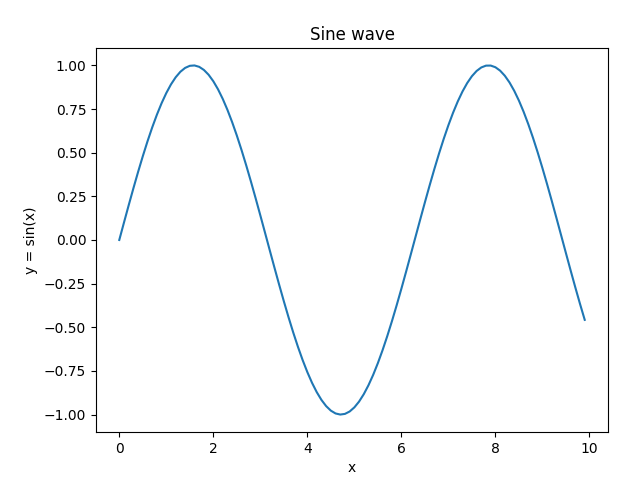