Matplotlib - Contour Plot
Contour plots also called level plots are a way to show a three-dimensional surface on a two-dimensional plane. It graphs two predictor variables X Y on the y-axis and a response variable Z as contours. These contours are sometimes called z-slices or iso-response values.
Contour plots are widely used to visualize density, altitudes or heights of the mountain as well as in the meteorological department. Due to such wide usage matplotlib.pyplot provides a method contour to make it easy to draw contour plots.
The matplotlib.pyplot.contour() and matplotlib.pyplot.contourf() functions are used to draw contour lines and filled contours, respectively.
Syntax
matplotlib.pyplot.contour([X, Y,] Z, [levels]) matplotlib.pyplot.contourf([X, Y,] Z, [levels])
Parameters
X, Y |
Optional. Specify 2-D numpy arrays with same shape as Z or 1-D arrays such that len(X) == M is the number of columns in Z and len(Y) == N is the number of rows in Z. |
Z |
Required. Specify the height values over which the contour is drawn. (M, N) array-like. |
levels |
Optional. Determines the number and positions of the contour lines / regions. int or array-like. |
Example: contour plot
In the example below, the contour() function to draw contour lines of an elliptical plane.
import matplotlib.pyplot as plt import numpy as np xlist = np.linspace(-5.0, 5.0, 100) ylist = ylist = np.linspace(-5.0, 5.0, 100) X, Y = np.meshgrid(xlist, ylist) #creating elliptical plane Z = (X**2)/4 + (Y**2)/9 fig, ax = plt.subplots() #drawing contour plot cb = ax.contour(X, Y, Z) #Adding a colorbar to the plot fig.colorbar(cb) ax.set_title('Contour Plot') ax.set_xlabel('x (cm)') ax.set_ylabel('y (cm)') plt.show()
The output of the above code will be:
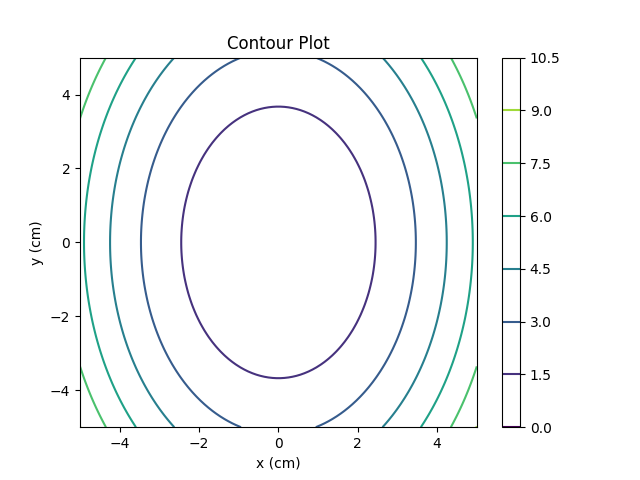
Example: filled contour plot
By using contourf() function, we can get filled contour plot.
import matplotlib.pyplot as plt import numpy as np xlist = np.linspace(-5.0, 5.0, 100) ylist = ylist = np.linspace(-5.0, 5.0, 100) X, Y = np.meshgrid(xlist, ylist) #creating hyperbolic plane Z = (X**2)/4 - (Y**2)/9 fig, ax = plt.subplots() #drawing filled contour plot cb = ax.contourf(X, Y, Z) #Adding a colorbar to the plot fig.colorbar(cb) ax.set_title('Filled Contour Plot') ax.set_xlabel('x (cm)') ax.set_ylabel('y (cm)') plt.show()
The output of the above code will be:
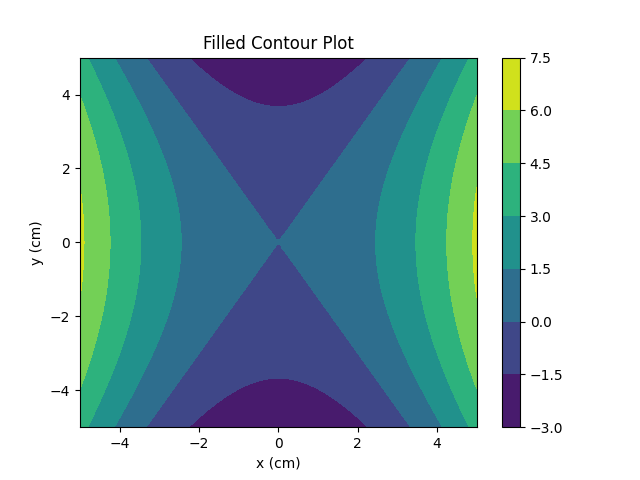