C++ - Standard Library Tutorial
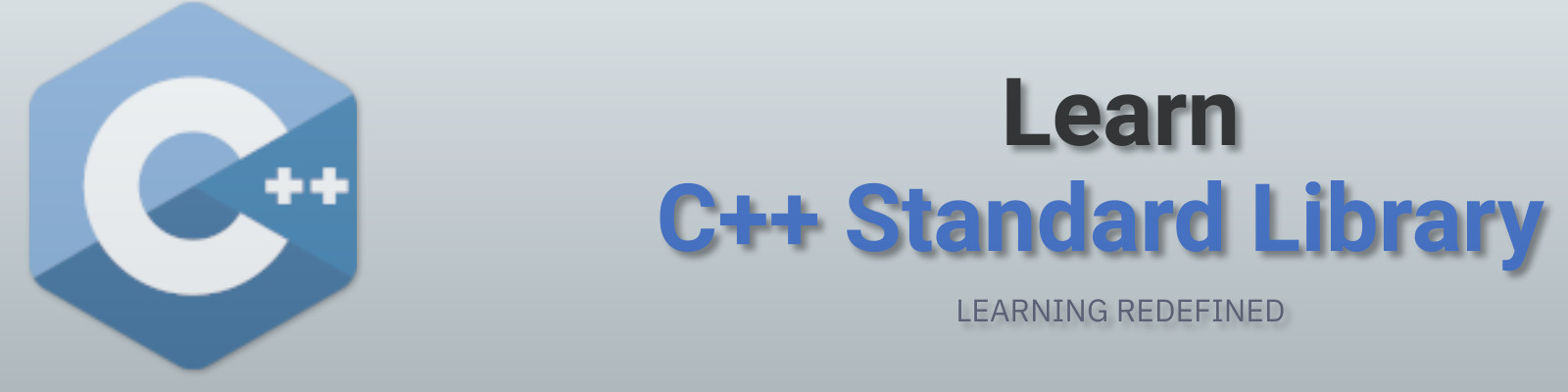
C++ is a middle-level and general-purpose programming language created by Bjarne Stroustrup starting in 1979 at Bell Labs. It is an extension to C programming with additional implementation of object-oriented programming (OOPs) concepts. It runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. C++ is the most widely used programming languages in application and system programming. There are various header files in C++ and vary depending on different compiler implementations. These header files includes the header files from C Standard Library, new C++ specific headers, and other important headers for the C++ Standard Template Library (STL).
About Tutorial
This tutorial is intended for students and professionals interested in studying basic and advanced concepts of C++ standard Library. This tutorial covers C built-in functions and library, and C++ STL Library. The C++ STL Library contains declaration, syntax and member functions of respective STL Library. We believe in learning by examples therefore each and every topic is explained with lots of examples that makes you learn the topic in a very easy way. Along with this, almost all examples can be executed online which provides better understanding of libraries and helps you to learn the concept faster. The classical example of calculating C++ array size is mentioned below for the illustration purpose.
#include <iostream> #include <array> using namespace std; int main (){ array<int, 100> Arr; cout<<"Array size is: "<<Arr.size(); return 0; }
The output of the above code will be:
Array size is: 100
Prerequisite
Before continuing with this tutorial, you should have basic understanding of C++ programming language. This section contains various types of examples which are used for reference and requires basic understanding of C++ language.