Java case Keyword
The Java case keyword is used to build a block of code in the switch statement. The switch expression is evaluated and matched with the cases. When the case matches, the following block of code is executed.
Syntax
switch (expression){ case 1: statement 1; break; case 2: statement 2; break; ... ... ... case N: statement N; break; }
Flow Diagram:
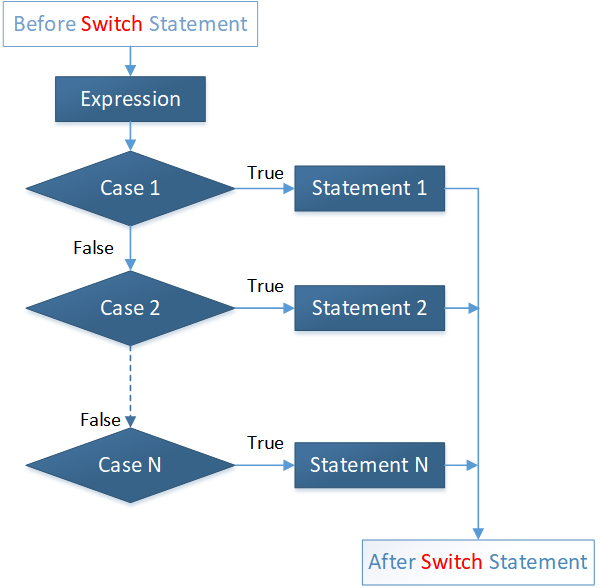
Example:
In the example below, the switch expression is a variable called i with value 2 which is matched against case values. When the case value matches with expression value, the following block of code is executed.
public class MyClass { public static void main(String[] args) { int i = 2; switch(i){ case 1: System.out.println("Red"); break; case 2: System.out.println("Blue"); break; case 3: System.out.println("Green"); break; } } }
The output of the above code will be:
Blue
❮ Java Keywords