Perl Math - sin() Function
The Perl Math sin() function returns trigonometric sine of an angle (angle should be in radians). In special cases it returns the following:
- If the argument is NaN or an infinity, then the result is NaN.
In the graph below, sin(x) vs x is plotted.
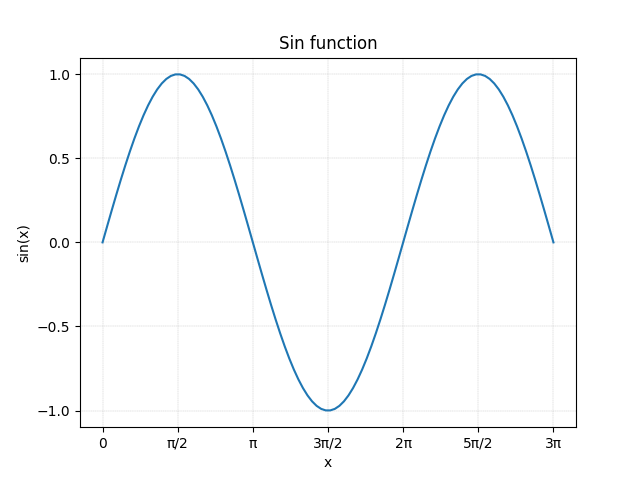
Syntax
sin(x)
Parameters
x |
Specify the angle in radian. |
Return Value
Returns the trigonometric sine of an angle.
Example:
In the example below, sin() function is used to find out the trigonometric sine of an angle.
use Math::Trig; print("sin(pi/6) = ".sin(pi/6)."\n"); print("sin(pi/4) = ".sin(pi/4)."\n"); print("sin(pi/3) = ".sin(pi/3)."\n"); print("sin(deg2rad(90)) = ".sin(deg2rad(90))."\n"); print("sin(deg2rad(120)) = ".sin(deg2rad(120))."\n"); print("sin(Inf) = ".sin(Inf)."\n"); print("sin(-Inf) = ".sin(-Inf)."\n"); print("sin(NaN) = ".sin(NaN)."\n");
The output of the above code will be:
sin(pi/6) = 0.5 sin(pi/4) = 0.707106781186547 sin(pi/3) = 0.866025403784439 sin(deg2rad(90)) = 1 sin(deg2rad(120)) = 0.866025403784439 sin(Inf) = NaN sin(-Inf) = NaN sin(NaN) = NaN
This function can also be used to calculate complex sine of a complex number z. It is a function on complex plane, and has no branch cuts. Mathematically, it can be expressed as:
Example:
In the example below, sin() function is used to find out the complex sine of the given number.
use Math::Complex; $z1 = 2 + 2*i; $z2 = 2; $z3 = 2*i; print("sin($z1) = ".sin($z1)."\n"); print("sin($z2) = ".sin($z2)."\n"); print("sin($z3) = ".sin($z3)."\n");
The output of the above code will be:
sin(2+2i) = 3.42095486111701-1.50930648532362i sin(2) = 0.909297426825682 sin(2i) = 3.62686040784702i
❮ Perl Math Functions