Scala - Math sin() Method
The Scala Math sin() method returns trigonometric sine of an angle (angle should be in radians). In special cases it returns the following:
- If the argument is NaN or an infinity, then the result is NaN.
In the graph below, sin(x) vs x is plotted.
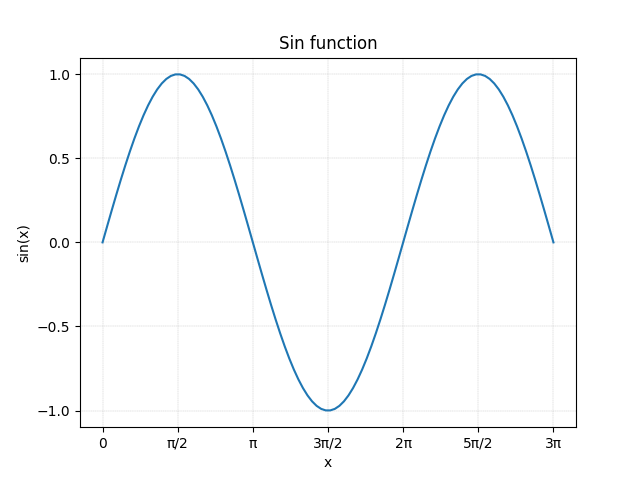
Syntax
def sin(x: Double): Double = scala.lang.Math.sin(x)
Parameters
x |
Specify the angle in radian. |
Return Value
Returns the trigonometric sine of the argument.
Exception
NA.
Example:
In the example below, sin() method is used to find out the trigonometric sine of the given angles.
import scala.math._ object MainObject { def main(args: Array[String]) { println(s"sin(Pi/6) = ${sin(Pi/6)}"); println(s"sin(Pi/4) = ${sin(Pi/4)}"); println(s"sin(Pi/3) = ${sin(Pi/3)}"); println(s"sin(Pi/2) = ${sin(Pi/2)}"); println(s"sin(Double.NaN) = ${sin(Double.NaN)}"); println(s"sin(2.0/0.0) = ${sin(2.0/0.0)}"); println("sin(Double.PositiveInfinity) = " + sin(Double.PositiveInfinity)); } }
The output of the above code will be:
sin(Pi/6) = 0.49999999999999994 sin(Pi/4) = 0.7071067811865475 sin(Pi/3) = 0.8660254037844386 sin(Pi/2) = 1.0 sin(Double.NaN) = NaN sin(2.0/0.0) = NaN sin(Double.PositiveInfinity) = NaN
❮ Scala - Math Methods