Rust - While Loop
A loop statement allows a program to execute a given block of codes repeatedly under a given condition. This enables a programmer to write programs in fewer lines of code and enhance the code readability. Rust has following types of loop to handle the looping requirements:
- while loop
- loop statement
- for loop
The While Loop
While loop allows a set of statements to be executed repeatedly as long as a specified condition is true. The While loop can be viewed as a repeating if statement.
Syntax
while (condition) { statements; }
Flow Diagram:
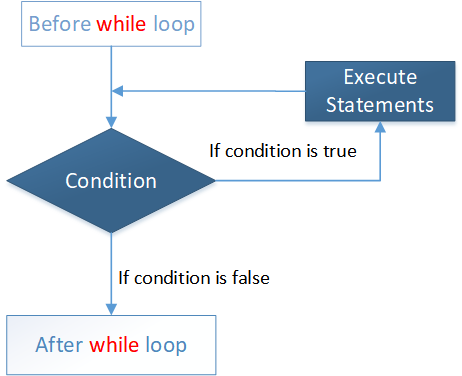
In below mentioned example, program uses while loop to sum all integers from 1 to 5.
fn main (){ let mut i = 1; let mut sum = 0; while (i < 6){ sum = sum + i; i = i + 1; } println!("Sum = {}", sum); }
The output of the above code will be:
Sum = 15
The loop Statement
One of the simplest way to create a loop in Rust is using the loop method. The loop method executes the block of code again and again until a manually intervene or break statement condition is fulfilled. It can be considered as a while(true) loop.
Syntax
loop { statements; }
In the example below, the program prints "Hello World!". It will keep on printing it until manually intervened.
fn main() { loop{ println!("Hello World!"); } }
The output of the above code will be:
Hello World! Hello World! Hello World! Hello World! Hello World! and so on...
To stop the loop, a break statement can be used. When the break condition is fulfilled, the program will get out of the loop. Consider the example below, where the program breaks the loop when the value of variable i becomes 5.
fn main() { let mut i = 0; loop{ i += 1; println!("value of i = {}", i); if(i == 5) { break; } } }
The output of the above code will be:
value of i = 1 value of i = 2 value of i = 3 value of i = 4 value of i = 5