C# Math - Cos() Method
The C# Cos() method returns trigonometric cosine of an angle (angle should be in radians). In special cases it returns the following:
- If the argument is NaN, negative infinity or positive infinity, the method returns NaN.
In the graph below, Cos(x) vs x is plotted.
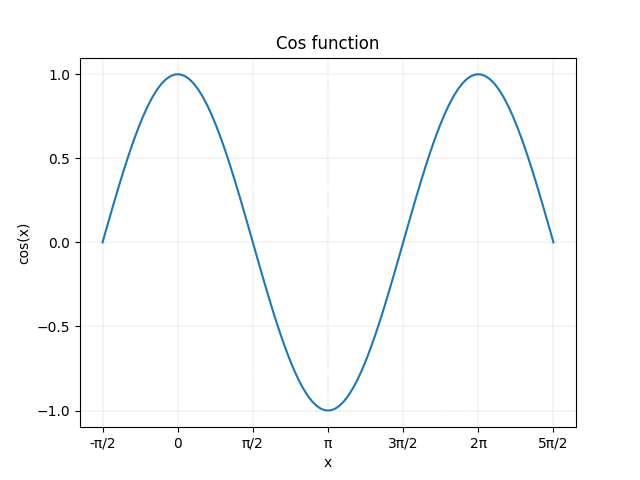
Syntax
public static double Cos (double arg);
Parameters
arg |
Specify the angle in radian. |
Return Value
Returns the trigonometric cosine of an angle.
Example:
In the example below, Cos() method is used to find out the trigonometric cosine of an angle.
using System; class MyProgram { static void Main(string[] args) { double pi = Math.PI; Console.WriteLine("Math.Cos(pi/6) = " + Math.Cos(pi/6)); Console.WriteLine("Math.Cos(pi/4) = " + Math.Cos(pi/4)); Console.WriteLine("Math.Cos(pi/3) = " + Math.Cos(pi/3)); Console.WriteLine("Math.Cos(Double.NaN) = " + Math.Cos(Double.NaN)); Console.WriteLine("Math.Cos(Double.NegativeInfinity) = " + Math.Cos(Double.NegativeInfinity)); Console.WriteLine("Math.Cos(Double.PositiveInfinity) = " + Math.Cos(Double.PositiveInfinity)); } }
The output of the above code will be:
Math.Cos(pi/6) = 0.866025403784439 Math.Cos(pi/4) = 0.707106781186548 Math.Cos(pi/3) = 0.5 Math.Cos(Double.NaN) = NaN Math.Cos(Double.NegativeInfinity) = NaN Math.Cos(Double.PositiveInfinity) = NaN
❮ C# Math Methods