JavaScript - If-Else Statements
If Statement
The If statement is used to execute a block of code when the condition is evaluated to be true. When the condition is evaluated to be false, the program will skip the if-code block.
Syntax
if(condition){ statements; }
Flow Diagram:
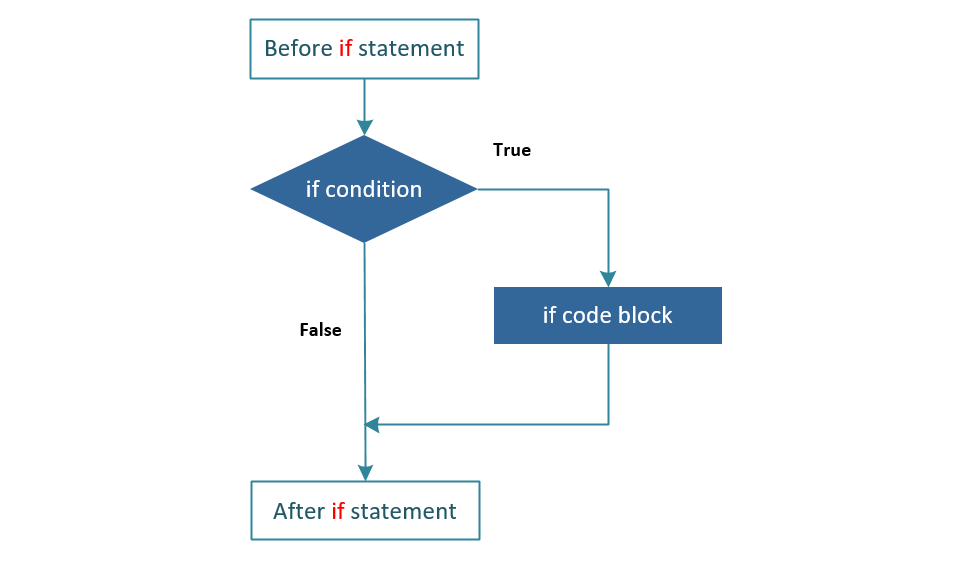
In the example below, the if code block is created which executes only when the variable i is divisible by 3.
var i = 15; var txt; if (i % 3 == 0){ txt = i + " is divisible by 3."; }
The output (value of txt) after running above script will be:
15 is divisible by 3.
If-else Statement
The else statement is always used with if statement. It is used to execute block of codes whenever if condition gives false result.
Syntax
if(condition){ statements; } else { statements; }
Flow Diagram:
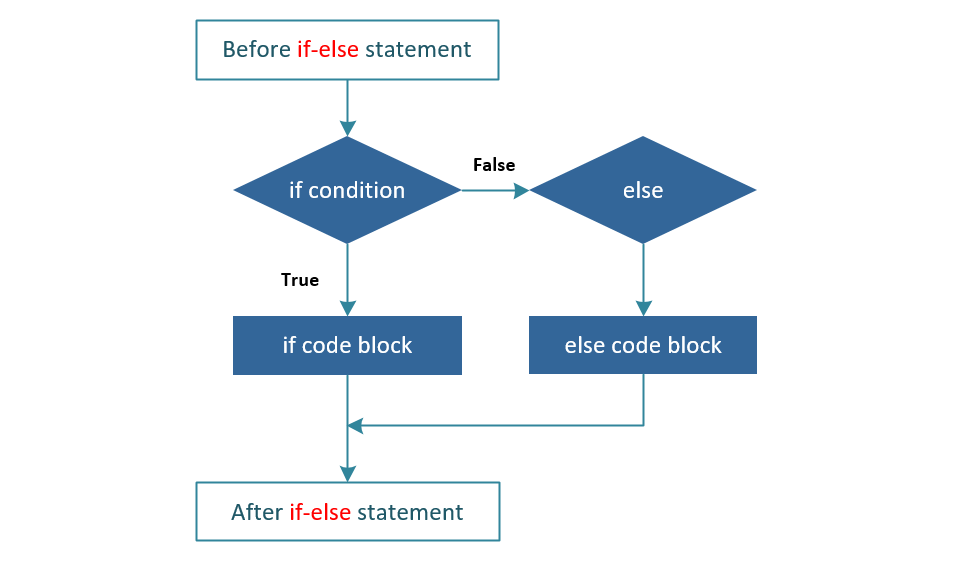
In the example below, if-else statement is used to perform time-based greeting.
var hour = new Date().getHours(); var greeting; //time-based gretting if(hour < 18) greeting = "Good Day!"; else greeting = "Good Evening!";
The output (value of greeting) after running above script will be:
else if Statement
For adding more conditions, else if statement in used. The program first checks if condition. When found false, then it checks else if conditions. If found false, then else code block is executed.
Syntax
if(condition){ statements; } else if(condition) { statements; } ... ... ... } else { statements; }
Flow Diagram:
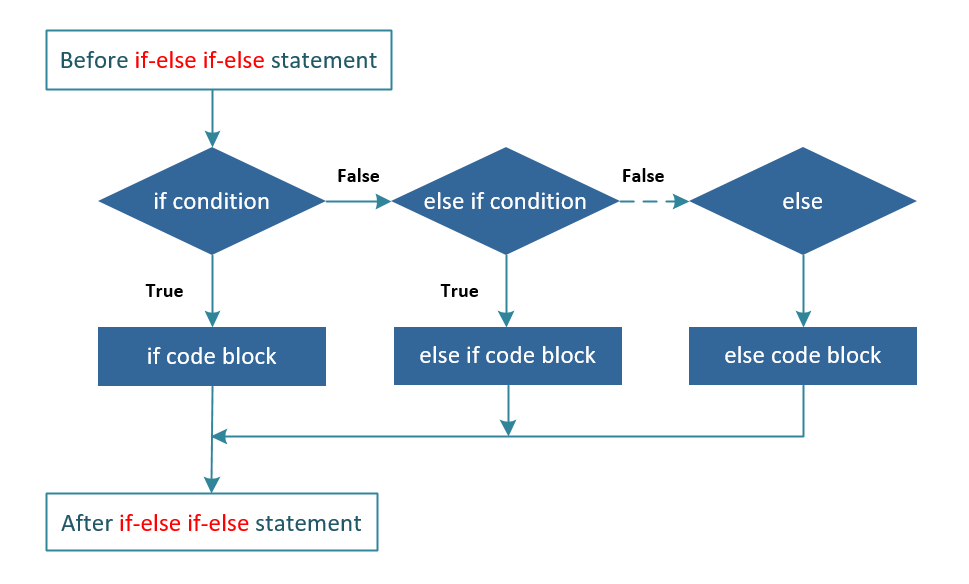
In the example below, if-elseif-else statement is used to perform time-based greeting.
var hour = new Date().getHours(); var greeting; //time-based gretting if(hour < 10) greeting = "Good Morning!"; else if(hour < 18) greeting = "Good Day!"; else greeting = "Good Evening!";
The output (value of greeting) after running above script will be: