JavaScript - While Loop
A loop statement allows a program to execute a given block of codes repeatedly under a given condition. This enables a programmer to write programs in fewer lines of code and enhance the code readability. JavaScript has following types of loop to handle the looping requirements:
- while loop
- do-while loop
- for loop
- for-in loop
- for-of loop
The While Loop
A while loop allows a set of statements to be executed repeatedly as long as a specified condition is true. The while loop can be viewed as a repeating if statement.
Syntax
while (condition) { statements; }
Flow Diagram:
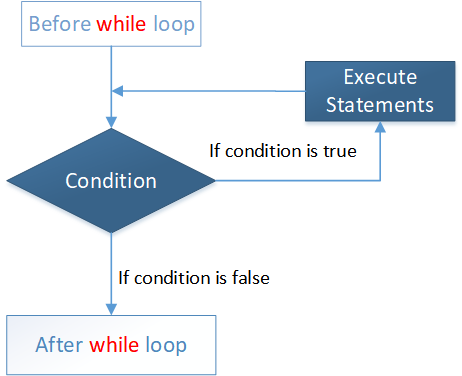
In below mentioned example, program uses while loop to sum all integers from 1 to 5.
var i = 0; var sum = 0; var txt; while (i <= 5) { sum = sum + i; i++; } txt = "Sum is: " + sum;
The output (value of txt) after running above script will be:
Sum is: 15
The Do-While Loop
The do-while loop is a variant of while loop which execute statements before checking the conditions. Therefore the do-while loop executes statements at least once.
Syntax
do { statements; } while (condition);
Flow Diagram:
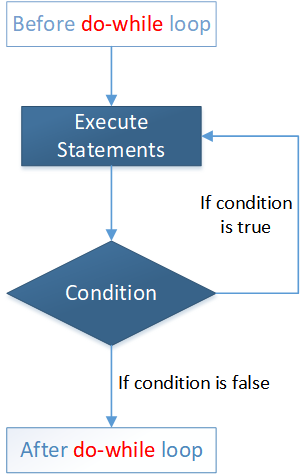
In the example below, even if the condition is not fulfilled, the do-while loop executes the statements once.
var i = 10; var sum = 0; var txt; do { sum = sum + i; i = i+1; } while (i <= 5); txt = "Sum is: " + sum;
The output (value of txt) after running above script will be:
Sum is: 10
While Loop over an array
In the example below an while loop is iterated over an array to collect the value of all elements.
var colors = ["Blue", "Red", "Green"]; var i = 0; var txt = ""; while (colors[i]) { txt = txt + colors[i] + "<br>"; i++; }
The output (value of txt) after running above script will be:
Blue Red Green