Pandas DataFrame - plot.area() function
An area plot displays quantitative data visually and stacked area plot displays the complete data with visualization of how each part makes up the whole. Each constituent of the stack area plot is stacked on top of each other.
The DataFrame.plot.area() functions makes an area plot.
Syntax
DataFrame.plot.area(x=None, y=None, stacked)
Parameters
x |
Optional. Specify coordinates for the x-axis using label or position. By default uses the index. |
y |
Optional. Specify ylabel or position indicating which column to plot. By default uses all columns. |
stacked |
Optional. Area plots are stacked by default. Set it to False to create a unstacked plot. |
Return Value
Returns matplotlib.axes.Axes or an ndarray with one matplotlib.axes.Axes per column when subplots=True.
Example: area plot of all columns
In the example below, a DataFrame Expenses is created. A area plot is created containing information about different types of monthly expenses (in stacked form).
import pandas as pd import numpy as np import matplotlib.pyplot as plt Expenses = pd.DataFrame({ "Housing": [550, 610, 590, 620, 650, 580, 550, 600, 710, 600, 580, 680], "Food": [200, 190, 220, 240, 200, 300, 240, 250, 220, 270, 200, 220], "Utility": [420, 450, 390, 320, 360, 450, 460, 380, 310, 430, 440, 390], "Insurance": [100, 120, 150, 90, 140, 160, 140, 120, 90, 140, 170, 120]}, index= ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"] ) #displaying the DataFrame print(Expenses,"\n") #creating the plot Expenses.plot.area() #displaying the plot plt.show()
The output of the above code will be:
Housing Food Utility Insurance Jan 550 200 420 100 Feb 610 190 450 120 Mar 590 220 390 150 Apr 620 240 320 90 May 650 200 360 140 Jun 580 300 450 160 Jul 550 240 460 140 Aug 600 250 380 120 Sep 710 220 310 90 Oct 600 270 430 140 Nov 580 200 440 170 Dec 680 220 390 120
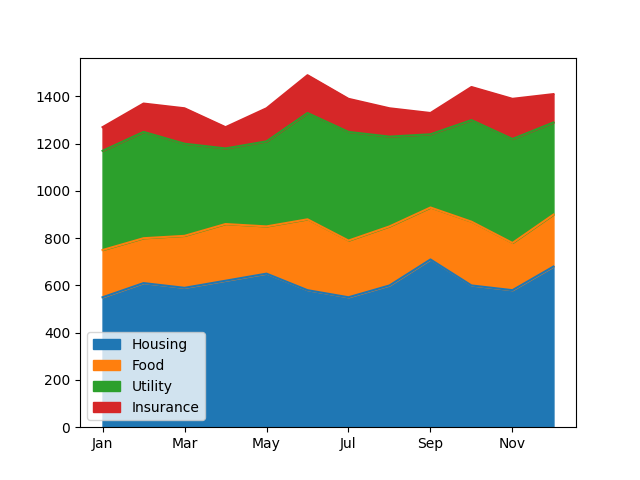
Example: Creating unstacked area plot
By using stacked=False parameter, an unstacked area plot can be created. consider the example below:
import pandas as pd import numpy as np import matplotlib.pyplot as plt Expenses = pd.DataFrame({ "Housing": [550, 610, 590, 620, 650, 580, 550, 600, 710, 600, 580, 680], "Food": [200, 190, 220, 240, 200, 300, 240, 250, 220, 270, 200, 220], "Utility": [420, 450, 390, 320, 360, 450, 460, 380, 310, 430, 440, 390], "Insurance": [100, 120, 150, 90, 140, 160, 140, 120, 90, 140, 170, 120]}, index= ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"] ) #displaying the DataFrame print(Expenses,"\n") #creating the plot Expenses.plot.area(stacked=False) #displaying the plot plt.show()
The output of the above code will be:
Housing Food Utility Insurance Jan 550 200 420 100 Feb 610 190 450 120 Mar 590 220 390 150 Apr 620 240 320 90 May 650 200 360 140 Jun 580 300 450 160 Jul 550 240 460 140 Aug 600 250 380 120 Sep 710 220 310 90 Oct 600 270 430 140 Nov 580 200 440 170 Dec 680 220 390 120
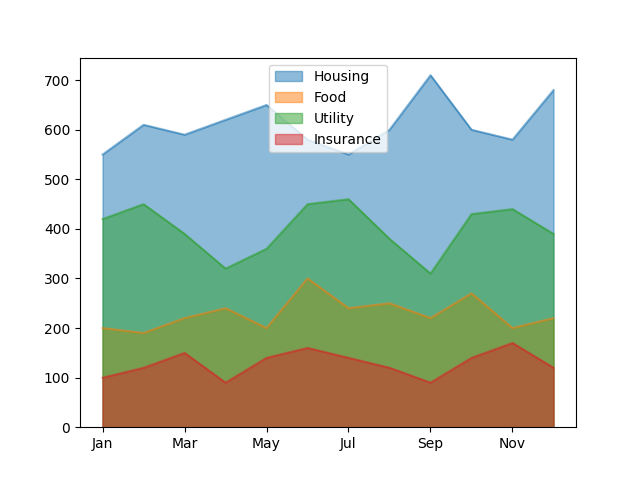
Example: using selected columns
In the example below, an area plot is created on selected columns.
import pandas as pd import numpy as np import matplotlib.pyplot as plt Expenses = pd.DataFrame({ "Housing": [550, 610, 590, 620, 650, 580, 550, 600, 710, 600, 580, 680], "Food": [200, 190, 220, 240, 200, 300, 240, 250, 220, 270, 200, 220], "Utility": [420, 450, 390, 320, 360, 450, 460, 380, 310, 430, 440, 390], "Insurance": [100, 120, 150, 90, 140, 160, 140, 120, 90, 140, 170, 120]}, index= ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"] ) #displaying the DataFrame print(Expenses,"\n") #creating the plot Expenses.plot.area(y=["Housing", "Utility"]) #displaying the plot plt.show()
The output of the above code will be:
Housing Food Utility Insurance Jan 550 200 420 100 Feb 610 190 450 120 Mar 590 220 390 150 Apr 620 240 320 90 May 650 200 360 140 Jun 580 300 450 160 Jul 550 240 460 140 Aug 600 250 380 120 Sep 710 220 310 90 Oct 600 270 430 140 Nov 580 200 440 170 Dec 680 220 390 120
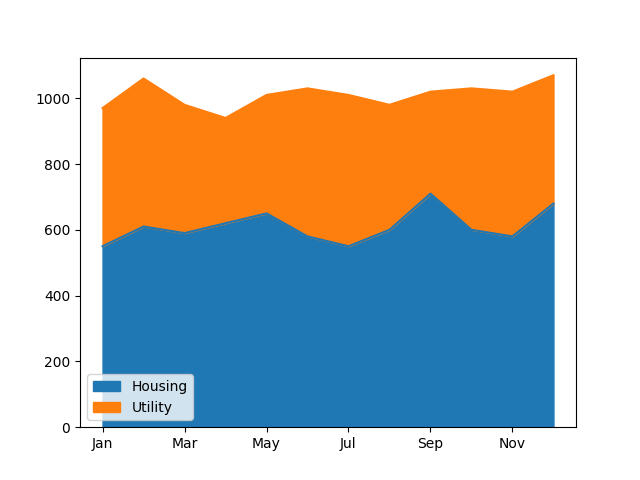
Example: Splitting the plot column wise
By using subplots=True parameter, we can split the area plot column wise. Consider the example below:
import pandas as pd import numpy as np import matplotlib.pyplot as plt Expenses = pd.DataFrame({ "Housing": [550, 610, 590, 620, 650, 580, 550, 600, 710, 600, 580, 680], "Food": [200, 190, 220, 240, 200, 300, 240, 250, 220, 270, 200, 220], "Utility": [420, 450, 390, 320, 360, 450, 460, 380, 310, 430, 440, 390], "Insurance": [100, 120, 150, 90, 140, 160, 140, 120, 90, 140, 170, 120]}, index= ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"] ) #displaying the DataFrame print(Expenses,"\n") #creating the plot Expenses.plot.area(y=["Housing", "Utility"], subplots=True) #displaying the plot plt.show()
The output of the above code will be:
Housing Food Utility Insurance Jan 550 200 420 100 Feb 610 190 450 120 Mar 590 220 390 150 Apr 620 240 320 90 May 650 200 360 140 Jun 580 300 450 160 Jul 550 240 460 140 Aug 600 250 380 120 Sep 710 220 310 90 Oct 600 270 430 140 Nov 580 200 440 170 Dec 680 220 390 120
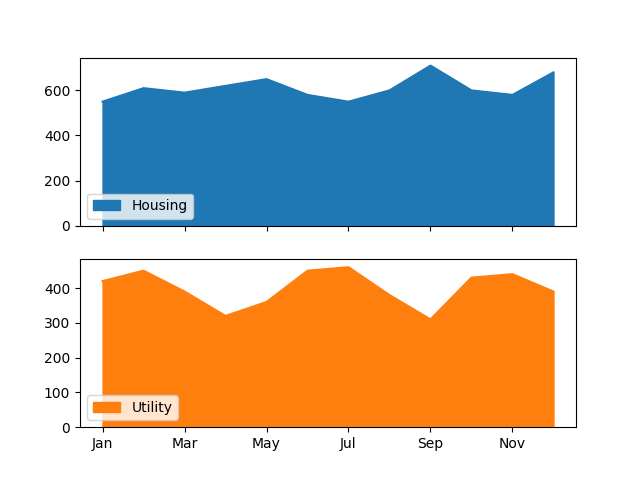
❮ Pandas DataFrame - Functions