PHP - Arrays
Arrays are used to store multiple values in a single variable. In PHP, an array can hold multiple data types. In PHP, there are three types of arrays:
- Indexed arrays - Arrays with numeric index
- Associative arrays - Arrays with named keys
- Multidimensional arrays - Arrays containing one or more arrays
In this section we will discuss about indexed array. Other types of arrays are discussed in later sections.
Create an Indexed Array
An indexed array can be created using array() keyword. It can be initialized at the time of creation by specifying all elements of the array within the array() keyword and separated by comma (,) or it can also be initialized later by specifying value using index number.
Alternatively, [] can also be used instead of array().
Syntax
//creating an empty array $MyArray = array(); $MyArray = []; //creating an array with initialization $MyArray = array(value1, value2, ...); $MyArray = [value1, value2, ...]; //initialization after creation of array $MyArray[index_number] = value;
Access element of an Indexed Array
An element of an indexed array can be accessed with it's index number. In PHP, the index number of an array starts with 0 as shown in the figure below:
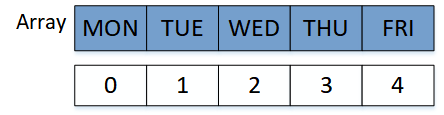
The example below describes how to access elements of an array using its index number.
<?php //creating an array with 3 elements $MyArray = array('MON', 'TUE', 'WED'); //using index number to assign values //to 4th and 5th elements of the array $MyArray[3] = 'THU'; $MyArray[4] = 'FRI'; for ($i = 0; $i < count($MyArray); $i++) { //access element using index number echo "Value at position=$i is $MyArray[$i] \n"; } ?>
The output of the above code will be:
Value at position=0 is MON Value at position=1 is TUE Value at position=2 is WED Value at position=3 is THU Value at position=4 is FRI
Modify elements of an Indexed Array
Any element of an indexed array can be changed using its index number and assigning a new value. Consider the example below:
<?php //creating an array with 5 elements $MyArray = array(10, 20, 30, 40, 50); //changing the value of element at index=1 $MyArray[1] = 1000; //displaying the array print_r($MyArray); ?>
The output of the above code will be:
Array ( [0] => 10 [1] => 1000 [2] => 30 [3] => 40 [4] => 50 )
1-based Indexed Array
To create a 1-based indexed array the following example can be used:
<?php $firstquarter = array(1 => 'JAN', 'FEB', 'MAR'); print_r($firstquarter); ?>
The output of the above code will be:
Array ( [1] => JAN [2] => FEB [3] => MAR )
Loop over Array
By using for loop, while loop or foreach loop, each elements of an indexed array can be accessed.
For Loop over Array
In the example below, for loop is used to access all elements of the given array.
<?php //creating an array with 5 elements $MyArray = array(10, 20, 30, 40, 50); for ($i = 0; $i < count($MyArray); $i++) { //access element using index number echo "\$MyArray[$i] = $MyArray[$i] \n"; } ?>
The output of the above code will be:
$MyArray[0] = 10 $MyArray[1] = 20 $MyArray[2] = 30 $MyArray[3] = 40 $MyArray[4] = 50
While Loop over Array
Similarly, while loop is also be used to access elements of the array.
<?php //creating an array with 5 elements $MyArray = array(10, 20, 30, 40, 50); $i = 0; while($i < count($MyArray)) { //access element using index number echo "\$MyArray[$i] = $MyArray[$i] \n"; $i++; } ?>
The output of the above code will be:
$MyArray[0] = 10 $MyArray[1] = 20 $MyArray[2] = 30 $MyArray[3] = 40 $MyArray[4] = 50
Foreach Loop over Array
In the example below, foreach loop is used to access all elements of an array.
<?php //creating an array with 5 elements $MyArray = array(10, 20, 30, 40, 50); echo "MyArray contains: "; foreach ($MyArray as $i) { echo $i." "; } ?>
The output of the above code will be:
MyArray contains: 10 20 30 40 50
Complete PHP Array Reference
For a complete reference of all PHP Array functions, see the complete PHP Arrays Reference.