PHP - Strings
PHP Strings
Strings are one of the most common data types in PHP. It is used for storing text. It can be created by enclosing characters either in single quotation marks or double quotation marks. It can be assigned to a variable using = sign.
$MyString = "Hello World!"; $MyString = 'Hello World!';
Access character of a String
A character (also called element) of a string can be accessed with it's index number. In PHP, index number starts with 0 in forward direction and -1 in backward direction. The figure below describes the indexing concept of a string.
String Indexing:
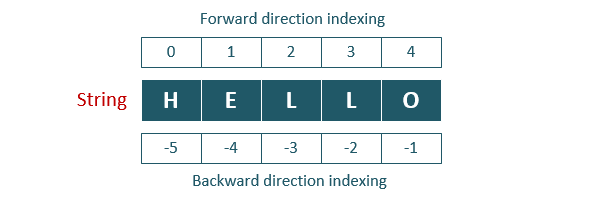
The example below describes how to access character of a string using its index number.
<?php $MyString = "HELLO"; echo $MyString[1]."\n"; echo $MyString[-1]."\n"; ?>
The output of the above code will be:
E O
String concatenation operator
To concatenate two string variables together, dot . operator can be used. See the example below:
<?php $str1 = "Hello"; $str2 = " World!"; $str3 = $str1 . $str2; echo $str3; ?>
The output of the above code will be:
Hello World!
String length
The strlen() function can be used in PHP to find out total number of characters in the given string.
<?php $MyString = "Hello World!"; echo strlen($MyString)."\n"; ?>
The output of the above code will be:
12
Word count of a string
The str_word_count() function is used to find out total number of words in the given string.
<?php $MyString = "Hello World"; echo str_word_count($MyString)."\n"; ?>
The output of the above code will be:
2
Reverse a string
A string in PHP can be reversed using strrev() function. Please note that it does not change the original string, only returns the reverse of the original string.
<?php $MyString = "Hello World"; echo strrev($MyString)."\n"; ?>
The output of the above code will be:
dlroW olleH
Check a text in the string
The PHP strpos() function is used to search the specified text in a given string. It returns the position of the text in the string. If no match is found, it returns 'FALSE'
<?php $MyString = "Hello World"; echo strpos($MyString, 'World')."\n"; ?>
The above code will give the following output:
6
Replace a text in the string
The PHP str_replace() function is used to replace the specified text with another text in a given string. In the example below 'World' is replaced by 'PHP'.
<?php $MyString = "Hello World"; echo str_replace('World', 'PHP', $MyString)."\n"; ?>
The above code will give the following output:
Hello PHP
Special characters in a string
The backslash \ escape character is used to convert special character like double quote, and new line, etc. into the string character. The below mentioned table describes special characters in PHP:
Escape Character | Result | Example |
---|---|---|
\" | " | "\"Hello\"" is converted into: "Hello" |
\$ | $ | "\$50" is converted into: $50 |
\\ | \ | "A\\C" is converted into: A\C |
\n | new line | "Hello\nJohn" is converted into: Hello John |
\t | Tab | "Hello\tMarry" is converted into: Hello Marry |
\r | Carriage return | "Hello\rJohn" is converted into: Hello John |
Complete PHP String Reference
For a complete reference of all PHP String functions, see the complete PHP String Reference.