Swift - Switch Statement
The Switch statement in Swift language is used to execute one of many code statements. It can be considered as group of If-else statements.
Syntax
switch (expression){ case 1: statement 1 break //optional case 2: statement 2 break //optional ... ... ... case N: statement N break //optional default: default statement }
The switch expression is evaluated and matched with the cases. When the case matches, the following block of code is executed.
Flow Diagram:
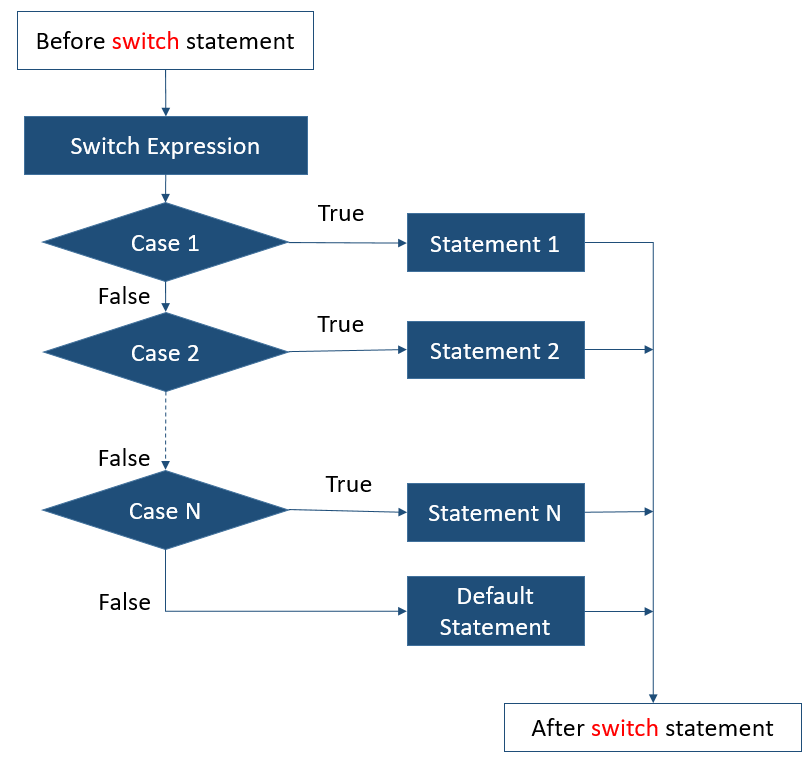
Example:
In the example below, the switch expression is a variable called i with value 2 which is matched against case values. When the case value matches with expression value, the following block of code is executed.
var i:Int = 2 switch(i){ case 1: print("Red") case 2: print("Blue") case 3: print("Green") default: print("No color is set.") }
The output of the above code will be:
Blue
default, break and fallthrough statements
In swift, a switch statement must include default statement. The break and fallthrough statements are optional here.
- Default Case: Default Statement is executed when there is no match between switch expression and test cases.
- Break Statement: Break statement is used to get out of the switch statement after a match is found. Swift don't fall through the bottom of each case and into the next one by default. Instead, the entire switch statement finishes its execution as soon as the first matching switch case is completed, without requiring an explicit break statement.
- Fallthrough Statement: fallthrough statement is used to fall through the subsequent case statements available below to matching case statement.
Example: Default statement
In the example below, the switch expression is a variable called i with value 10 which is matched against case values. As there is no case that matches with value 10, hence default block of code gets executed.
var i:Int = 10 switch(i){ case 1: print("Red") case 2: print("Blue") case 3: print("Green") default: print("No color is set.") }
The output of the above code will be:
No color is set.
Please note that, the default statement must be placed at the bottom of the switch statement.
Example: Break and Fallthrough statements
Consider the example below, where fallthrough statement is used to fall through the subsequent case statements available below to matching case statement.
var i:Int = 3 switch(i){ case 1: print("Red") case 2: print("Blue") case 3: print("Green") fallthrough case 4: print("White") fallthrough case 5: print("Black") break case 6: print("Yellow") default: print("No color is set.") }
The output of the above code will be:
Green White Black
Common code blocks
There are instances where same code block is required in multiple cases.
Example:
In the example below, same code block is shared for different switch cases.
var i:Int = 10 switch(i){ case 1: print("Red") case 2, 10: print("Blue") case 3, 4, 5: print("Green") default: print("No color is set.") }
The output of the above code will be:
Blue
Adding more conditions in case statements
Swift gives the flexibility to add more conditions in case statements of a switch expression. This can be achieved by using where expression.
Example:
In the example below, the second, third and fourth case statements, all use where expressions to match ranges of numbers.
var i:Int = 50 switch(i){ case 1: print("Red") case let i where i == 2 || i == 10: print("Blue") case let i where i == 3 || i == 4 || i == 5: print("Green") case let i where i > 10: print("Yellow") default: print("No color is set.") }
The output of the above code will be:
Yellow