Swift - While Loop
A loop statement allows a program to execute a given block of codes repeatedly under a given condition. This enables a programmer to write programs in fewer lines of code and enhance the code readability. Swift has following types of loop to handle the looping requirements:
- while loop
- repeat-while loop
- for-in loop
The While Loop
While loop allows a set of statements to be executed repeatedly as long as a specified condition is true. The While loop can be viewed as a repeating if statement.
Syntax
while (condition) { statements }
Flow Diagram:
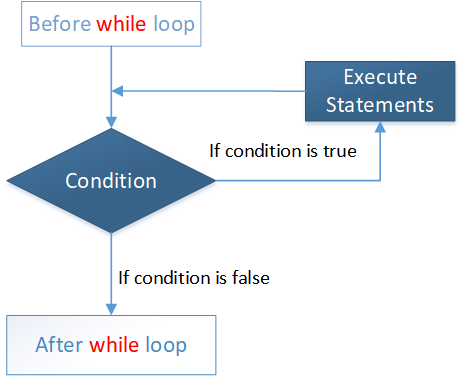
In below mentioned example, program uses while loop to sum all integers from 1 to 5.
var i = 1 var sum = 0 while (i < 6) { sum = sum + i i = i + 1 } print(sum)
The output of the above code will be:
15
Consider one more example, where iterating variable i is printed in each iteration.
var i:Int = 100 while (i <= 1000) { print("Value of i = \(i)") i = i + 100 }
The output of the above code will be:
Value of i = 100 Value of i = 200 Value of i = 300 Value of i = 400 Value of i = 500 Value of i = 600 Value of i = 700 Value of i = 800 Value of i = 900 Value of i = 1000