C - For Loop
The for loop executes a set of statements in each iteration. Whenever, number of iteration is known, for loop is preferred over while loop.
Syntax
for(initialization(s); condition(s); counter_update(s);){ statements; }
- Initialization(s): Variable(s) is initialized in this section and executed for one time only.
- Condition(s): Condition(s) is defined in this section and executed at the start of loop everytime.
- Counter Update(s): Loop counter(s) is updated in this section and executed at the end of loop everytime.
Flow Diagram:
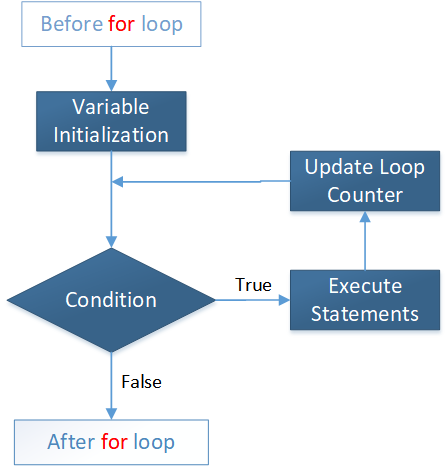
Example:
In the example below, the program continue to print variable called i from value 1 to 5. After that, it exits from the for loop.
#include <stdio.h> int main (){ for(int i = 1; i <= 5; i++){ printf("%i\n", i); } return 0; }
The output of the above code will be:
1 2 3 4 5
Example
Multiple initializations, condition checks and loop counter updates can be performed in a single for loop. In the example below, two variable called i and j are initialized, multiple conditions are checked and multiple variables are updated in a single for loop.
#include <stdio.h> int main (){ for(int i = 1, j = 100; i <= 5 || j <= 800; i++, j = j + 100){ printf("i=%i, j=%i\n",i,j); } return 0; }
The output of the above code will be:
i=1, j=100 i=2, j=200 i=3, j=300 i=4, j=400 i=5, j=500 i=6, j=600 i=7, j=700 i=8, j=800