C - While Loop
A loop statement allows a program to execute a given block of codes repeatedly under a given condition. This enables a programmer to write programs in fewer lines of code and enhance the code readability. C has following types of loop to handle the looping requirements:
- while loop
- do-while loop
- for loop
The While Loop
While loop allows a set of statements to be executed repeatedly as long as a specified condition is true. The While loop can be viewed as a repeating if statement.
Syntax
while (condition) { statements; }
Flow Diagram:
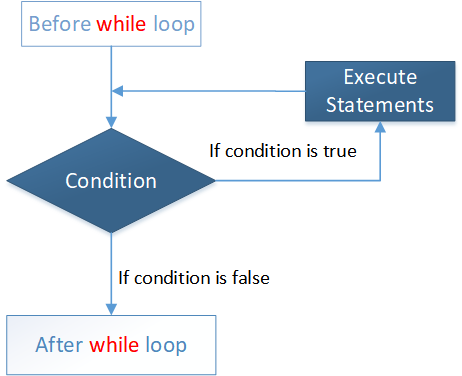
In below mentioned example, program uses while loop to sum all integers from 1 to 5.
#include <stdio.h> int main (){ int i = 1; int sum = 0; while (i < 6){ sum = sum + i; i = i+1; } printf("%i",sum); return 0; }
The output of the above code will be:
15
The Do-While Loop
The Do-While loop in C is a variant of while loop which execute statements before checking the conditions. Therefore the Do-While loop executes statements at least once.
Syntax
do { statements; } while (condition);
Flow Diagram:
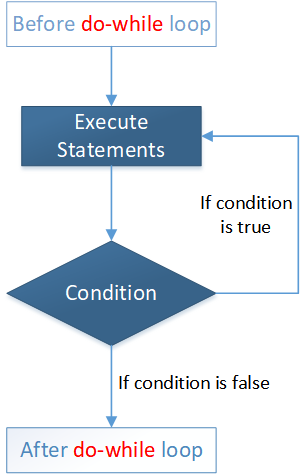
In the example below, even if the condition is not fulfilled, the do-while loop executes the statements once.
#include <stdio.h> int main (){ int i = 10; int sum = 0; do { sum = sum + i; i = i+1; } while (i < 6); printf("%i",sum); return 0; }
The output of the above code will be:
10