Python - Strings
Python Strings
Strings are one of the most common data types in Python. It is used for storing text. It can be created by enclosing characters either in single quotation marks or double quotation marks. It can be assigned to a variable using = sign.
MyString = "Hello World!" MyString = 'Hello World!'
Python Multi-line Strings
Multi-line string can be created by enclosing the block either in three single quotation marks or three double quotation marks.
MyString = """Python programming""" MyString = '''Python programming'''
Access character of a String
A character (also called element) of a string can be accessed with it's index number. In Python, index number starts with 0 in forward direction and -1 in backward direction. The figure below describes the indexing concept of a string.
String Indexing:
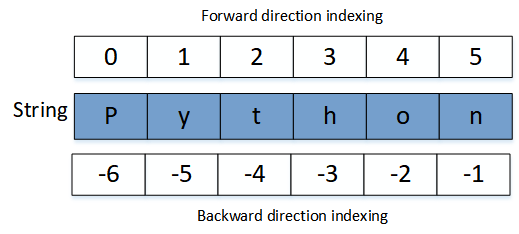
The example below describes how to access character of a string using its index number.
MyString = 'Python' #forward indexing print(MyString[0]) #backward indexing print(MyString[-1])
The output of the above code will be:
P n
Access range of characters of a String
Range of characters of a string can be selected using statement like [startIndex : endIndex] where end_index is excluded. If start_index and end_index are not mentioned then it takes first and last index numbers of the string respectively.
MyString = 'Learn Python' print(MyString[0:5]) print(MyString[-12:-7],"\n") print(MyString[6:]) print(MyString[:-7],"\n") print(MyString[:])
The output of the above code will be:
Learn Learn Python Learn Learn Python
String Length
The len() function can be used to find out total number of characters in the string.
MyString = 'Learn Python' print(len(MyString))
The output of the above code will be:
12
Check a character(s) in the String
If control statement is used to check whether the string contains specified character(s) or not.
MyString = 'I am learning Python programming.' if 'programming' in MyString: print('Yes, It is present in the string.') else: print('No, It is not present in the string.')
The above code will give the following output:
Yes, It is present in the string.
String Concatenation
Two strings can be joined using + operator.
text_1 = 'Learn' text_2 = 'Python' MyString = text_1 + text_2 print(MyString) MyString = text_1 + " " + text_2 print(MyString)
The above code will give the following output:
LearnPython Learn Python
String format() Method
Strings can not be added with numbers by using + operator. For combining string with a number, format method is used. A user can pass parameter(s) in format() method, which is further placed into its respective placeholder { }. This method can take unlimited number of parameters. Along with this, an index number (starts with 0) can also be used with placeholders. See the example below:
in_time = 9 out_time = 18 MyString = "I will reach office at {} hrs and leave office at {} hrs." print(MyString.format(in_time, out_time)) in_time = 9 out_time = 18 MyString = "I will reach office at {1} hrs and leave office at {0} hrs." print(MyString.format(out_time, in_time))
The above code will give the following output:
I will reach office at 9 hrs and leave office at 18 hrs. I will reach office at 9 hrs and leave office at 18 hrs.
String Methods
Python has number of string methods. Here, few very common string functions are discussed.
- lower(): Returns string in lowercase
- upper(): Returns string in uppercase
- strip(): Removes whitespaces from start and end of the string
- replace(): replace specified character(s) with another specified character(s)
- split(): Split the string by specified separator and returns substrings in a list.
- count(): Returns number of occurrence of specified character(s) in the string
Example: lower(), upper() and strip() String Methods
The example below shows how to use lower(), upper() and strip() methods in python.
MyString = "Learn Python" print(MyString.lower()) print(MyString.upper()) MyString = " Learn Python " print(MyString.strip())
The above code will give the following output:
learn python LEARN PYTHON Learn Python
Example: replace(), split() and count() String Methods
In the example below replace(), split() and count() methods is used while working with a string.
MyString = "Learn Python" print(MyString.replace("Python", "C++")) MyString = "Learning Python is fun" print(MyString.split(" ")) MyString = "This is Python programming." print(MyString.count('is'))
The above code will give the following output:
Learn C++ ['Learning', 'Python', 'is', 'fun'] 2