Python - While Loop
A loop statement allows a program to execute a given block of codes repeatedly under a given condition. This enables a programmer to write programs in fewer lines of code and enhance the code readability. Python has following types of loop to handle the looping requirements:
- while loop
- for loop
The While Loop
The While loop allows a set of statements to be executed repeatedly as long as a specified condition is true. It can be viewed as a repeating if statement. A while loop is preferred over for loop whenever number of iteration is not known beforehand.
Syntax
while condition: statements
Flow Diagram:
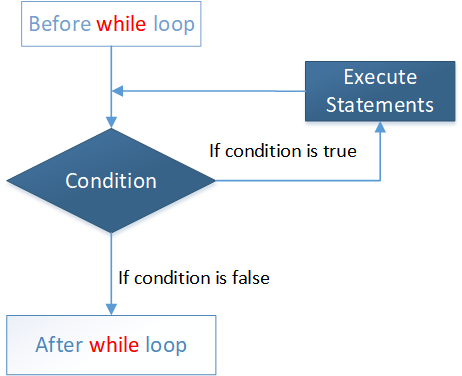
In below mentioned example, program uses while loop to sum all integers from 1 to 5.
sum = 0 i = 1 while (i < 6): sum = sum + i i = i+1 print(sum)
The output of the above code will be:
15
While loop with else statement
The else block can be used with while loop also. The else block is executed when condition check in while loop returns false.
Syntax
while condition: statements else: statements
In below mentioned example, else code block is executed after exiting from while loop.
i = 1 while (i < 6): print(i) i = i + 1 else: print('loop exhausted.')
The output of the above code will be:
1 2 3 4 5 loop exhausted.
However, when program exits from while loop using break statements, it ignores the else block.
i = 1 while (i < 6): print(i) i = i + 1 if (i==4): break else: print('loop exhausted.')
The output of the above code will be:
1 2 3