NumPy - Uniform Distribution
Uniform Distribution describes an experiment where there is an random outcome that lies between certain bounds. The bounds of the outcome are defined by the parameters, a and b, which are the minimum and maximum values. All intervals of the same length on the distribution has equal probability.
The probability density function (pdf) of uniform distribution is defined as:
Where, a and b are lower and upper boundaries of output interval respectively.
An uniform distribution has mean (a+b)/2 and variance (b-a)2/12.
The cumulative distribution function (cdf) evaluated at x, is the probability that the random variable (X) will take a value less than or equal to x. The cdf of uniform distribution is defined as:
The NumPy random.uniform() function returns random samples from a uniform distribution.
Syntax
numpy.random.uniform(low=0.0, high=1.0, size=None)
Parameters
low |
Optional. Specify the lower boundary of the output interval. float or array_like of floats. Default is 0.0. |
high |
Optional. Specify the upper boundary of the output interval. float or array_like of floats. Must be non-negative. Default is 1.0. |
size |
Optional. Specify output shape. int or tuple of ints. If the given shape is (m, n, k), then m * n * k samples are drawn. If size is None (default), a single value is returned if low and high are both scalars. Otherwise, np.broadcast(low, high).size samples are drawn. |
Return Value
Returns samples from the parameterized uniform distribution. ndarray or scalar.
Example: Values from uniform distribution
In the example below, random.uniform() function is used to create a matrix of given shape containing random values drawn from specified uniform distribution.
import numpy as np size = (5,3) sample = np.random.uniform(0, 1, size) print(sample)
The possible output of the above code could be:
[[0.93041354 0.50856806 0.46275855] [0.31003963 0.10581335 0.64064579] [0.58998769 0.44103259 0.44828118] [0.22591024 0.10336945 0.74814414] [0.95073832 0.70598443 0.4166474 ]]
Plotting uniform distribution
Example: Density plot
Matplotlib is a plotting library for the Python which can be used to plot the probability density function (pdf) of uniform distribution using hist() function.
import matplotlib.pyplot as plt import numpy as np #fixing the seed for reproducibility #of the result np.random.seed(10) size = 10000 #drawing 10000 sample from #uniform distribution sample = np.random.uniform(0, 1, size) bin = np.arange(-1,2,0.05) plt.hist(sample, bins=bin, edgecolor='blue') plt.title("Uniform Distribution") plt.show()
The output of the above code will be:
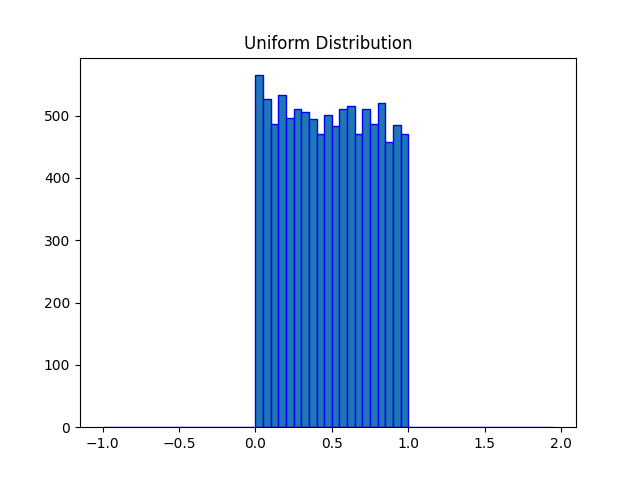
Example: Comparing pdfs
Multiple probability density functions can be compared graphically using Seaborn kdeplot() function. In the example below, pdf of three uniform distributions (each with different low and high) are compared.
import numpy as np import matplotlib.pyplot as plt import seaborn as sns #fixing the seed for reproducibility #of the result np.random.seed(10) size = 10000 #plotting 10000 sample from #different uniform distribution sns.kdeplot(np.random.uniform(0, 1, size)) sns.kdeplot(np.random.uniform(0, 2, size)) sns.kdeplot(np.random.uniform(0, 3, size)) plt.legend(["a = 0, b = 1", "a = 0, b = 2", "a = 0, b = 3"]) plt.show()
The output of the above code will be:
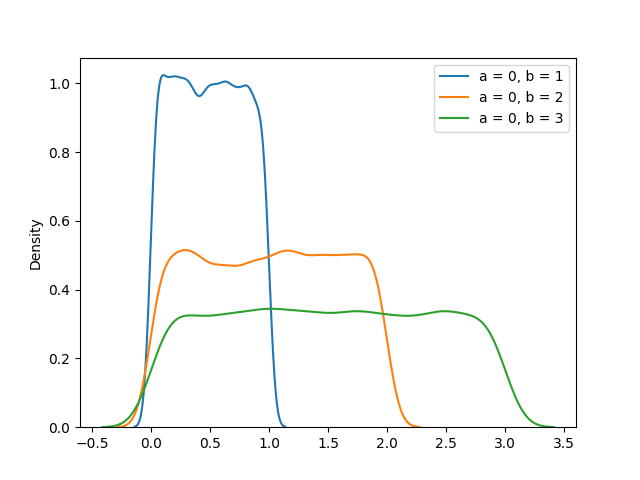
Example: Comparing cdfs
Multiple cumulative distribution functions can be compared graphically using Seaborn ecdfplot() function. In the example below, cdf of three uniform distributions (each with different low and high) are compared.
import numpy as np import matplotlib.pyplot as plt import seaborn as sns #fixing the seed for reproducibility #of the result np.random.seed(10) size = 1000 #plotting 1000 sample from #different uniform distribution sns.ecdfplot(np.random.uniform(0, 1, size)) sns.ecdfplot(np.random.uniform(0, 2, size)) sns.ecdfplot(np.random.uniform(0, 3, size)) plt.legend(["a = 0, b = 1", "a = 0, b = 2", "a = 0, b = 3"]) plt.show()
The output of the above code will be:
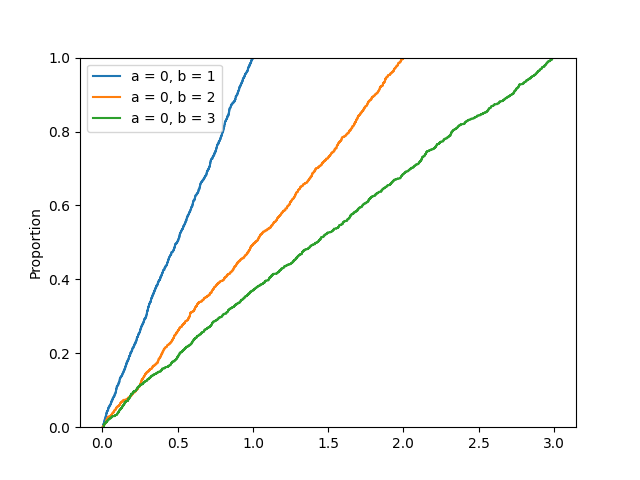