R - Switch Statement
The Switch statement in R language is used to execute one of many code statements. It can be considered as group of If-else statements.
Syntax
switch (expression, case1, case2, case3, ...)
The switch expression is evaluated and matched with the cases. When the case matches, the following block of code is executed. The following rules apply to a R switch statement:
- If the value of expression is not a character string it is coerced to integer.
- If the value of the integer is between 1 and number of cases then the corresponding element of case condition is evaluated and returned.
- If expression evaluates to a character string then that string is matched (exactly) to the listed cases.
- For multiple matches, the first match element will be used.
- No Default argument is available in R switch case.
- If there is no match, there is a unnamed element of ... its value is returned.
Flow Diagram:
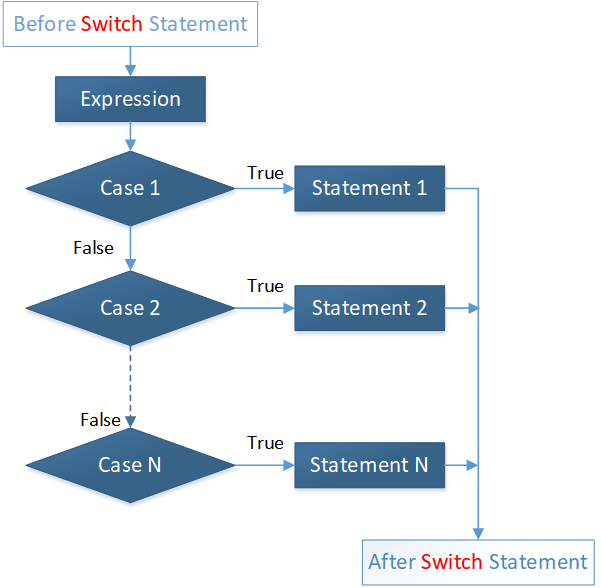
Example:
In the example below, the switch expression is a variable called i with value 3. As the value of the switch expression is an integer hence the third element of case condition is evaluated and returned.
i <- 3 val <- switch( i, "Red", "Blue", "Green", "Yellow", "Black", "White" ) print(val)
The output of the above code will be:
[1] "Green"
Example:
Consider one more example where the switch expression is a string variable. In this case the switch expression is matched against listed cases and corresponding section is evaluated and returned.
i <- "d" val <- switch( i, "a" = "Red", "b" = "Blue", "c" = "Green", "d" = "Yellow", "e" = "Black", "f" = "White" ) print(val)
The output of the above code will be:
[1] "Yellow"