R - While Loop
A loop statement allows a program to execute a given block of codes repeatedly under a given condition. This enables a programmer to write programs in fewer lines of code and enhance the code readability. R has following types of loop to handle the looping requirements:
- while loop
- for loop
- repeat loop
The While Loop
The While loop allows a set of statements to be executed repeatedly as long as a specified condition is true. It can be viewed as a repeating if statement. A while loop is preferred over for loop whenever number of iteration is not known beforehand.
Syntax
while (condition) { statements }
Flow Diagram:
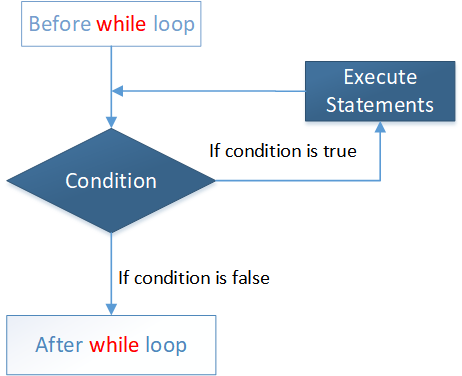
In below mentioned example, program uses while loop to sum all integers from 1 to 5.
sum <- 0 i <- 1 while (i < 6){ sum <- sum + i i <- i+1 } print(sum)
The output of the above code will be:
[1] 15
next statement
By using next statement, the program skips the loop whenever condition is met, and brings the program to the start of loop. Please consider the example below.
i <- 1 #skips the loop for odd value of i while (i <= 10) { if(i %% 2 == 1) { i <- i + 1 next } print(i) i <- i + 1 }
The output of the above code will be:
[1] 2 [1] 4 [1] 6 [1] 8 [1] 10
break statement
By using break statement, the program get out of the for loop when condition is met. Please consider the example below.
i <- 1 #get out of the loop when i == 5 while (i <= 10) { if(i == 5) break print(i) i <- i + 1 }
The output of the above code will be:
[1] 1 [1] 2 [1] 3 [1] 4